Vue Form Validation with Vuelidate
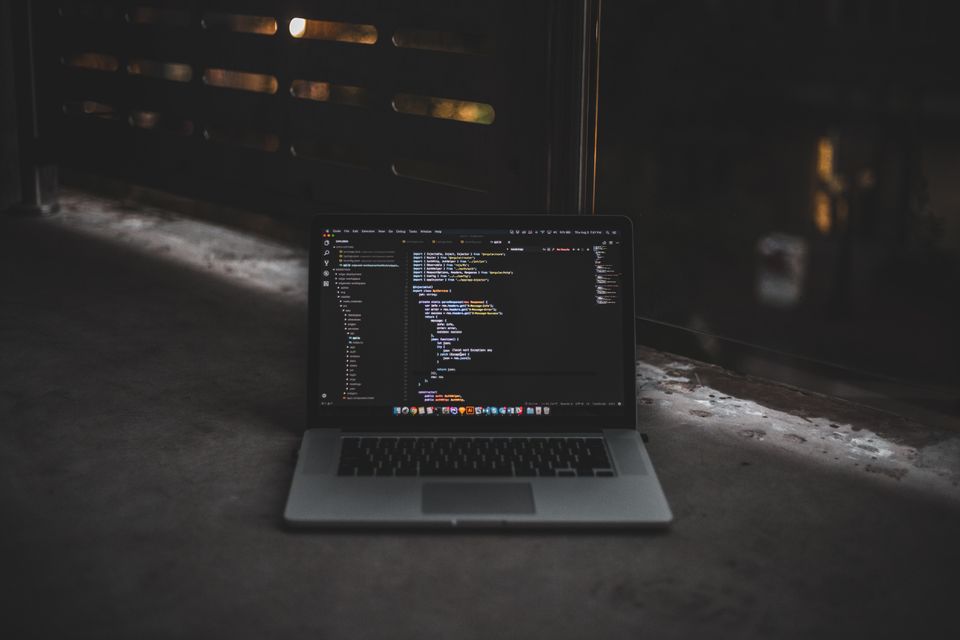
Introduction
Form validation using basic Vue is straightforward. The problem comes with the lack of different validation checks and dynamic checking. Instead of writing my form validation functions, I decided to use Vuelidate.
What is vuelidate
Vuelidate is a simple, lightweight model-based validation for Vue.js. It is model-based, decoupled from templates, and has a collection of validations. It's considered the standard validation library for Vue.js.
How do I install Vuelidate
1) Install the package via npm
npm install vuelidate --save
2) Import the library and use as a Vue plugin.
import Vue from 'vue'
import Vuelidate from 'vuelidate'
Vue.use(Vuelidate)
How do I use Vuelidate
<template>
<form @submit="handleSubmit">
<input type="text" v-model="name" />
<input type="submit">
</form>
</template>
<script>
import { required, minLength } from 'vuelidate/lib/validators'
export default {
data() {
return {
name: '',
age: 0
}
},
validations: {
name: {
required,
minLength: minLength(4)
}
},
methods: {
handleSubmit(e) {
this.$v.$touch() // we check the form to see if it's valid
if (this.$v.$invalid) return; // if any of the validations fail, we don't move on
console.log('yay your form is successful')
}
}
}
</script>
As you can see from above, we have a simple form. We use vuelidate and its libraries to require the name model. Required
means the name cannot be an empty string. We also validate the minimum length of the name
is at least 4 characters. This validation happens after the user clicks on the submit button. In the handleSubmit
method, we use $v.$touch
to check the validation of the model.
If the name
model is not validated, then we don't move on, usually this is where we have some sort of error message.
How to use requiredIf with props
We can also create our custom validation. My goal to create a conditional validation based on its props. Vuelidate has a validator requiredIf
which can make the field optionally or conditionally required. We can combine this with the prop by passing a function to requiredIf
.
prop: {
active: {
type: Boolean,
default: true
}
},
validations: {
field: {
required: requiredIf(function () {
return this.active // return true if this field is required
})
}
}