How to check if an array is empty in javascript
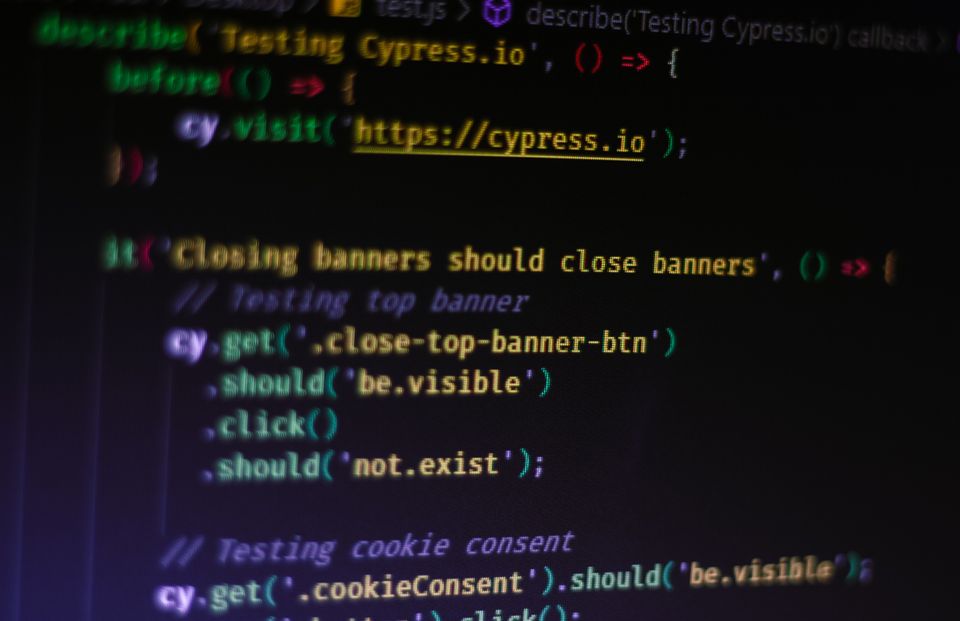
How do we check to see how many elements are in an array?
We can use the length
property of the type Array
.
const arr = [1,2,3]
console.log(arr.length) // 3
We see that the printing out arr.length
equals 3 because there are 3 elements in our array.
Empty would mean we should not have any elements in our array at all.
const emptyArr = []
console.log(arr.length) // 0
In order to check if our array is empty, we would need to see if the length equals 0
. We can write an if statement similar to below
if (arrayName.length === 0) {
// if my array is empty then do this
}
Resources
Array.length
The length property of an object which is an instance of type Array sets or returns the number of elements in that array. The value is an unsigned, 32-bit integer that is always numerically greater than the highest index in the array.
