How To Create a Vue Country Code Dropdown
Introduction
I wanted to create a Vue dropdown component that allows users to select a country. Ideally, I would prefer to use a package that provides country codes with the country selection. With over 200+ countries, I’d rather not do it by hand.
What are country codes?
Country codes are short alphabetic or numeric geographic codes, similar to state initials, except for countries.
Country code package
I found a npm package i18n-iso-countries
which provides us the ISO 3166-1 code in Alpha-2, Alpha-3, and Numeric codes. We can always double-check the [official country codes] through Wikipedia.
Installation
Install with npm: npm install i18n-iso-countries --save
To use it in a browser environment, we need to register the language.
// Support English languages.
countries.registerLocale(require("i18n-iso-countries/langs/en.json"));
Creating the select input
Let’s create the country select dropdown with selected as a data variable. Selection options are generally in an Array full of Objects in label, value pairs.
<template>
<select v-model="selected">
<option v-for="option in options" :value="option.value">
{{ option.label }}
</option>
</select>
</template>
<script>
export default {
name: 'CountrySelection',
data () {
return {
selected: null,
options: []
}
}
}
</script>
Importing the country codes
Let’s import the i18n-iso-countries
package and register the English locale.
<template>
...
</template>
<script>
const countries = require('i18n-iso-countries')
countries.registerLocale(require('i18n-iso-countries/langs/en.json'))
export default {
name: 'CountrySelection',
data () {
...
}
}
</script>
Instead of using a data option variable, I’m going to map through the countries and create an array as computed.
<template>
...
</template>
<script>
const countries = require('i18n-iso-countries')
countries.registerLocale(require('i18n-iso-countries/langs/en.json'))
export default {
name: 'CountrySelection',
computed: {
countries () {
const list = countries.getNames('en', { select: 'official' })
return Object.keys(list).map((key) => ({ value: key, label: list[key] }))
}
},
data () {
return {
selected: null
}
}
}
</script>
Creating a Vue Country Code Select
Now, we can update the options to iterate through the countries
computed property.
<template>
<select v-model="selected">
<option v-for="country in countries" :value="country.value">
{{ country.label }}
</option>
</select>
</template>
<script>
const countries = require('i18n-iso-countries')
countries.registerLocale(require('i18n-iso-countries/langs/en.json'))
export default {
name: 'CountrySelection',
computed: {
countries () {
const list = countries.getNames('en', { select: 'official' })
return Object.keys(list).map((key) => ({ value: key, label: list[key] }))
}
},
data () {
return {
selected: null
}
}
}
</script>
Conclusion
Using i18n-iso-countries
, we created a Vue selection component that provides us with the ISO codes of each country. This is a great addition to any Vue form for international users.
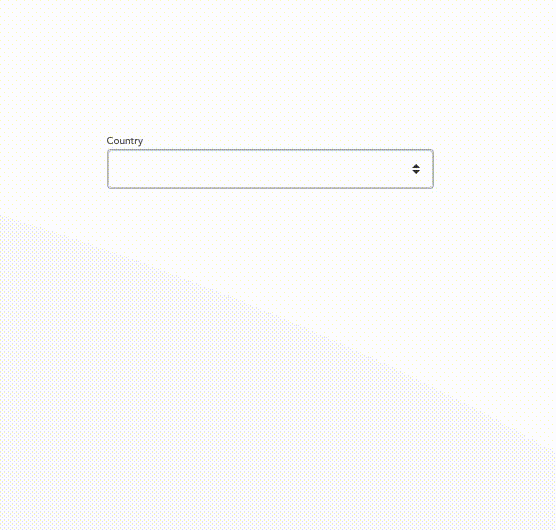